[ OpenCV ] Basic 4 - 이미지 회전 (Rotate)
반응형
Image Rotate
이미지를 특정 각도로 회전하는 방법에 대해 알아보겠습니다. 이미지를 회전할 때에는 회전할 지점을 지정해야 합니다. 대부분의 경우는 이미지의 중심을 기준으로 회전을 하지만 임의의 지점을 지정할 수도 있습니다.
Import packages
import cv2 import numpy as np import imutils import matplotlib.pyplot as plt
Jupyter Notebook 및 Google Colab에서 이미지를 표시할 수 있도록 Function으로 정의
def img_show(title='image', img=None, figsize=(8 ,5)): plt.figure(figsize=figsize) if type(img) == list: if type(title) == list: titles = title else: titles = [] for i in range(len(img)): titles.append(title) for i in range(len(img)): if len(img[i].shape) <= 2: rgbImg = cv2.cvtColor(img[i], cv2.COLOR_GRAY2RGB) else: rgbImg = cv2.cvtColor(img[i], cv2.COLOR_BGR2RGB) plt.subplot(1, len(img), i + 1), plt.imshow(rgbImg) plt.title(titles[i]) plt.xticks([]), plt.yticks([]) plt.show() else: if len(img.shape) < 3: rgbImg = cv2.cvtColor(img, cv2.COLOR_GRAY2RGB) else: rgbImg = cv2.cvtColor(img, cv2.COLOR_BGR2RGB) plt.imshow(rgbImg) plt.title(title) plt.xticks([]), plt.yticks([]) plt.show()
Load Image
cv2_image = cv2.imread('asset/images/test_image.jpg', cv2.IMREAD_COLOR)
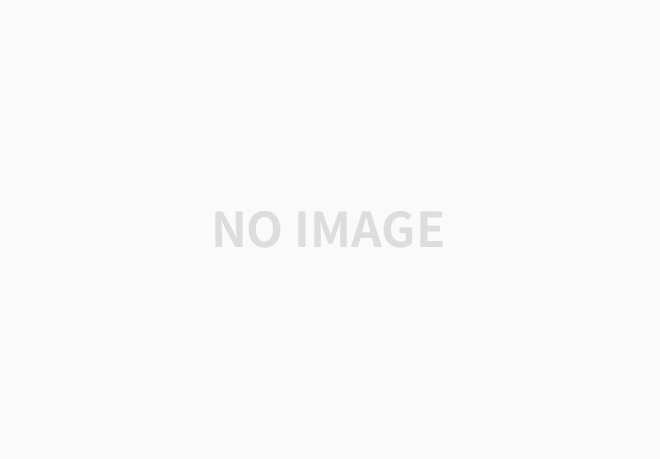
Rotate
이미지의 크기를 잡고 이미지의 중심을 계산합니다. 이 중심을 기준으로 이미지를 45도 회전 하거나 -90도 회전하는 예시입니다.
# 이미지의 크기를 잡고 이미지의 중심을 계산합니다. (h, w) = cv2_image.shape[:2] (cX, cY) = (w // 2, h // 2) # 이미지의 중심을 중심으로 이미지를 45도 회전합니다. M = cv2.getRotationMatrix2D((cX, cY), 45, 1.0) rotated_45 = cv2.warpAffine(cv2_image, M, (w, h)) # 이미지를 중심으로 -90도 회전 M = cv2.getRotationMatrix2D((cX, cY), -90, 1.0) rotated_90 = cv2.warpAffine(cv2_image, M, (w, h)) img_show(["Rotated by 45 Degrees", "Rotated by -90 Degrees"], [rotated_45, rotated_90])
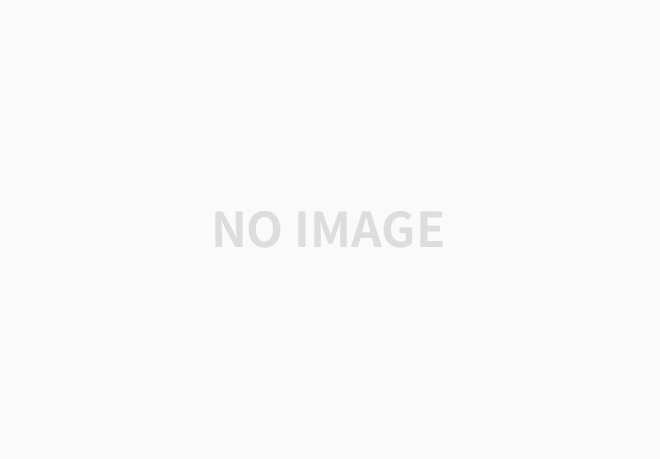
이미지를 이동 할 때 행렬을 정의했던 것처럼 이미지를 회전 시에도 행렬을 정의하여 이용 할 수 있습니다. NumPy를 사용하여 수동으로 행렬을 구성하는 대신 cv2.getRotationMatrix2D메서드를 사용합니다.
첫번째 인수는 이미지를 회전하는 지점입니다. (이 경우 이미지의 중심 cX과 위치 cY) 두번째 인수는 각도 입니다. 세번째 인수는 이미지 크기입니다. (예를들어 1.0 이면 원본사이즈, 2.0 이면 이미지 크기가 두배가 됩니다.)
M = cv2.getRotationMatrix2D((10, 10), 45, 1.0) rotated = cv2.warpAffine(cv2_image, M, (w, h)) img_show("Rotated by Arbitrary Point", rotated)
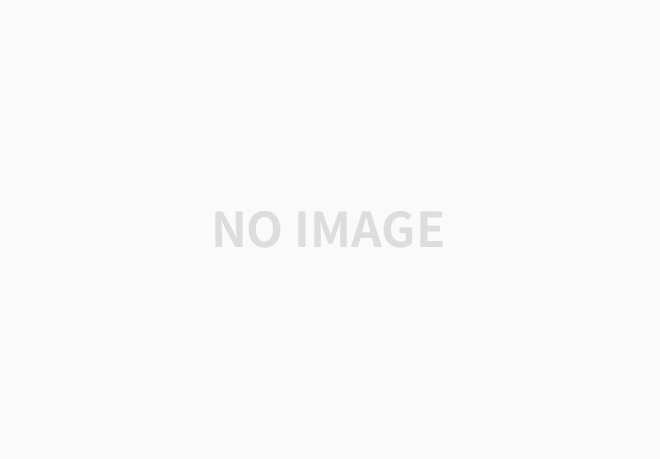
Rotate images using imutils
imutils 함수를 사용하여 이미지를 180도 회전합니다.
rotated = imutils.rotate(cv2_image, 180) img_show("Rotated by 180 Degrees", rotated)
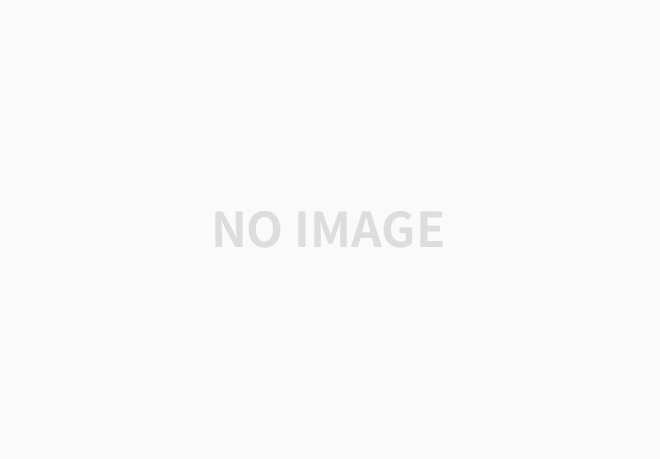
imutils.rotate_bound을 사용하면 회전 시 이미지 전체가 그안에 맞 도록 이미지 배열을 자동으로 확장합니다.
rotated = imutils.rotate_bound(cv2_image, -33) img_show("Rotated Without Cropping", rotated)
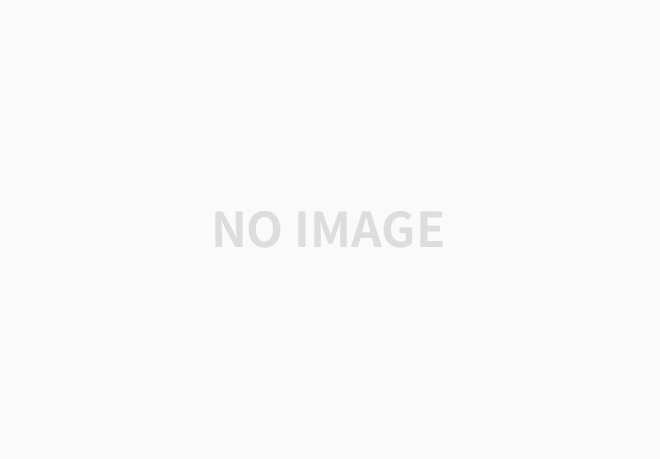
반응형
'Tech & Development > Image Processing' 카테고리의 다른 글
[ OpenCV ] Basic 6 - 이미지 크기조정 (Resize) (0) | 2022.03.02 |
---|---|
[ OpenCV ] Basic 5 - 이미지 상하, 좌우 대칭 (Flip) (0) | 2022.03.02 |
[ OpenCV ] Basic 3 - 이미지 이동 (Shift) (0) | 2022.03.02 |
[ OpenCV ] Basic 2 - 기본 그리기 (선, 점, 사각형, 원) (0) | 2022.01.10 |
[ OpenCV ] Basic 1 - 픽셀(Pixel)값 가져오고 설정하기 (0) | 2022.01.10 |
댓글
이 글 공유하기
다른 글
-
[ OpenCV ] Basic 6 - 이미지 크기조정 (Resize)
[ OpenCV ] Basic 6 - 이미지 크기조정 (Resize)
2022.03.02Image Resize 고해상도의 이미지는 사람의 눈으로 보기에는 좋아 보이지만 컴퓨터가 연산하거나 이미지 파이프라인 처리에는 좋지 않을 수 있습니다. 이미지가 큰 경우 더 많은 데이터가 필요하기 때문에 알고리즘이 데이터를 처리하는 데 오래 걸리기 때문입니다. 이런 고해상도 이미지는 매우 디테일하지만, 컴퓨터 비전/이미지 처리 관점에서 볼 때 이러한 디테일한 사항보다는 이미지의 구조적 구성 요소가 더 중요하기 때문에 고해상도의 이미지를 다운샘플링하여 더 빠르고 정확하게 실행될 수 있도록 합니다. Import packages import cv2 import imutils import matplotlib.pyplot as plt Jupyter Notebook 및 Google Colab에서 이미지를 표시하고 … -
[ OpenCV ] Basic 5 - 이미지 상하, 좌우 대칭 (Flip)
[ OpenCV ] Basic 5 - 이미지 상하, 좌우 대칭 (Flip)
2022.03.02Image Flip x축 또는 y축을 가로질러 이미지를 뒤집는 방법을 소개하겠습니다. 다시말하자면 이미지를 수평 또는 수직을 기준으로 미러링 이미지로 변환하는 것입니다. Flip은 이미지 데이터를 부풀리기 위해서 사용되기도 합니다. Import packages import cv2 import numpy as np import imutils import matplotlib.pyplot as plt Jupyter Notebook 및 Google Colab에서 이미지를 표시할 수 있도록 Function으로 정의 def img_show(title='image', img=None, figsize=(8 ,5)): plt.figure(figsize=figsize) if type(img) == list: if type… -
[ OpenCV ] Basic 3 - 이미지 이동 (Shift)
[ OpenCV ] Basic 3 - 이미지 이동 (Shift)
2022.03.02Image Shift OpenCV를 이용하여 이미지를 이동하는 방법에 대해 설명드리겠습니다. imutils를 이용하여 간단하게 이미지를 이동 할 수 있지만 기본이 되는 affine transformation matrix (cv2.warpAffine)을 사용하는 방법을 포함하여 설명드리도록 하겠습니다. affine transformation matrix 이라고 하는 2 x 3 행렬을 정의해야 합니다. 이 행렬은 이미지가 왼쪽 또는 오른쪽으로 몇 픽셀을 이동될 것인지, 또는 이미지를 위 또는 아래로 몇 픽셀이 이동할 것인지 정의합니다. 음수 t{x} 값은 이미지를 왼쪽 으로 이동 양수 t{x} 값은 이미지를 오른쪽 으로 이동 음수 t{y} 값은 이미지 를 위로 이동 양수 t{y} 값은 이미지 를 아래로 이동… -
[ OpenCV ] Basic 2 - 기본 그리기 (선, 점, 사각형, 원)
[ OpenCV ] Basic 2 - 기본 그리기 (선, 점, 사각형, 원)
2022.01.10Drawing with OpenCV OpenCV 기본 그리기 기능을 사용하는 방법을 설명드리겠습니다. OpenCV에는 불규칙한 모양의 다각형을 포함하여 다양한 모양을 그리는 데 사용할 수 있는 여러 그리기 기능이 있지만 가장 일반적인 3가지 OpenCV 그리기 기능은 다음과 같습니다. cv2.line : 지정된 (x, y) 좌표 에서 시작 하여 다른 (x, y) 좌표 에서 끝나는 이미지에 선을 그립니다. cv2.circle : 중심 (x, y) 좌표와 제공된 반지름으로 지정된 이미지에 원을 그립니다. cv2.rectangle : 왼쪽 위 모서리와 오른쪽 아래 모서리 (x, y) 좌표로 지정된 이미지에 직사각형을 그립니다. Import packages import cv2 import numpy as np …
댓글을 사용할 수 없습니다.