[OpenCV] Basic 9 - 이미지 경계선 검출 (Edge Detection)
경계선 검출(Edge Detection)은 이미지에서 물체의 윤곽선을 찾아내는 방법입니다. 이미지 내에서 밝기가 급격히 변하는 부분을 탐지하여 객체의 형태를 추출할 수 있습니다. OpenCV에서는 여러 가지 경계선 검출 기법을 제공합니다. 대표적으로 Canny Edge Detection과 Sobel Filter가 많이 사용됩니다.
Import packages
import cv2 import numpy as np import matplotlib.pyplot as plt
Function to Display Images in Jupyter Notebook
Jupyter Notebook 및 Google Colab에서 이미지를 표시할 수 있도록 Function으로 정의합니다.
def img_show(title='image', img=None, figsize=(8, 5)): plt.figure(figsize=figsize) if type(img) == list: titles = title if type(title) == list else [title] * len(img) for i in range(len(img)): rgbImg = cv2.cvtColor(img[i], cv2.COLOR_GRAY2RGB) if len(img[i].shape) <= 2 else cv2.cvtColor(img[i], cv2.COLOR_BGR2RGB) plt.subplot(1, len(img), i + 1) plt.imshow(rgbImg) plt.title(titles[i]) plt.xticks([]), plt.yticks([]) plt.show() else: rgbImg = cv2.cvtColor(img, cv2.COLOR_GRAY2RGB) if len(img.shape) < 3 else cv2.cvtColor(img, cv2.COLOR_BGR2RGB) plt.imshow(rgbImg) plt.title(title) plt.xticks([]), plt.yticks([]) plt.show()
Load Image
이미지를 불러옵니다.
cv2_image = cv2.imread('asset/images/test_image.jpg', cv2.IMREAD_COLOR) gray_image = cv2.cvtColor(cv2_image, cv2.COLOR_BGR2GRAY)
1. Canny Edge Detection
Canny Edge Detection은 가장 널리 사용되는 경계선 검출 기법 중 하나로, 이미지의 노이즈 제거 후 경계선을 찾아냅니다. threshold1
과 threshold2
값에 따라 검출된 경계선의 민감도가 달라집니다.
canny_edges = cv2.Canny(gray_image, 100, 200) img_show("Canny Edge Detection", canny_edges)
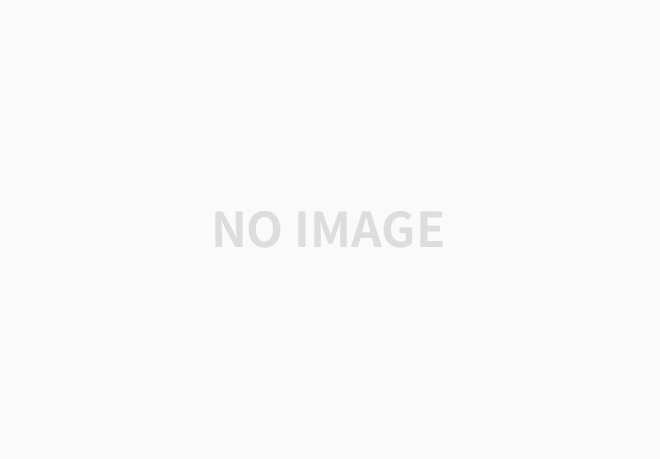
2. Sobel Filter
Sobel Filter는 이미지의 밝기 변화 방향을 계산하여 경계선을 검출하는 기법입니다. X축과 Y축 각각에 대해 경계선을 검출한 후, 합성하여 전체 경계선을 생성합니다.
- Sobel X: X축 방향의 경계선 검출
- Sobel Y: Y축 방향의 경계선 검출
sobel_x = cv2.Sobel(gray_image, cv2.CV_64F, 1, 0, ksize=3) sobel_y = cv2.Sobel(gray_image, cv2.CV_64F, 0, 1, ksize=3) sobel_x = cv2.convertScaleAbs(sobel_x) sobel_y = cv2.convertScaleAbs(sobel_y) sobel_edges = cv2.addWeighted(sobel_x, 0.5, sobel_y, 0.5, 0) img_show(["Sobel X", "Sobel Y", "Sobel Magnitude"], [sobel_x, sobel_y, sobel_edges])
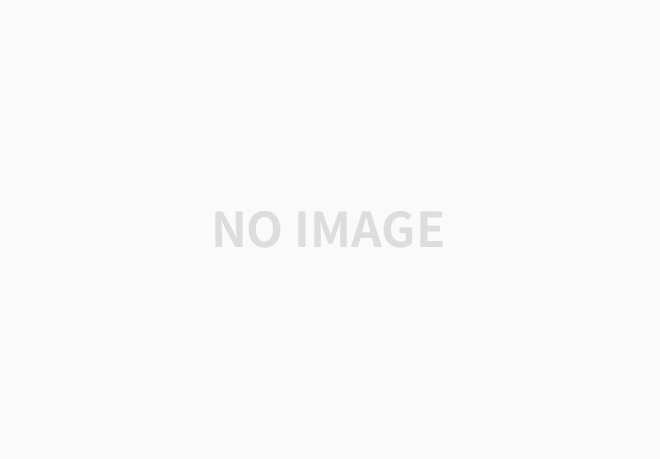
3. Laplacian Edge Detection
Laplacian 필터는 이미지에서 고차 미분을 사용하여 경계선을 검출합니다. Sobel Filter보다 더 날카로운 경계선을 생성할 수 있습니다.
laplacian_edges = cv2.Laplacian(gray_image, cv2.CV_64F) laplacian_edges = cv2.convertScaleAbs(laplacian_edges) # 8-bit로 변환 img_show("Laplacian Edge Detection", laplacian_edges)
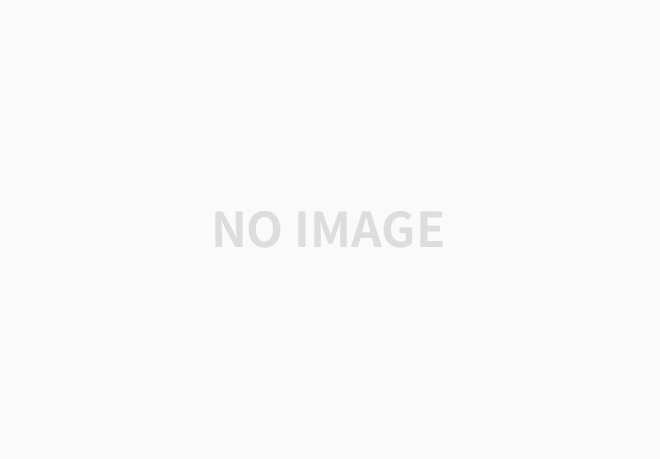
4. Combined Edge Detection
Canny, Sobel, Laplacian 등을 조합하여 복합적인 경계선 검출을 할 수 있습니다. 예를 들어, Sobel 필터로 X, Y 방향을 각각 검출하고, Canny Edge로 최종 경계선을 강조할 수 있습니다.
combined_edges = cv2.Canny(cv2.convertScaleAbs(sobel_edges), 50, 150) img_show("Combined Edge Detection", combined_edges)
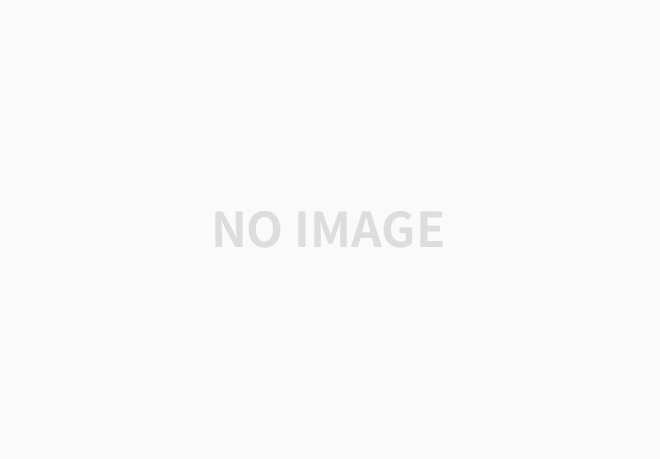
위와 같이 OpenCV를 사용하여 다양한 방법으로 경계선을 검출할 수 있습니다. 각 방법은 고유의 특성이 있으므로, 이미지의 특징과 목표에 따라 적절한 기법을 선택하는 것이 좋습니다.
'Tech & Development > Image Processing' 카테고리의 다른 글
[OpenCV] Basic 11 - 이미지 히스토그램 (Histogram) 분석 (0) | 2024.11.19 |
---|---|
[OpenCV] Basic 10 - 이미지 색상 변환 (Color Conversion) (1) | 2024.11.16 |
[OpenCV] Basic 8 - 이미지 블러 처리 (Blur) (0) | 2024.11.14 |
[ OpenCV ] Basic 7 - 이미지 자르기 (Crop) (0) | 2022.11.21 |
[ OpenCV ] Basic 6 - 이미지 크기조정 (Resize) (0) | 2022.03.02 |
댓글
이 글 공유하기
다른 글
-
[OpenCV] Basic 11 - 이미지 히스토그램 (Histogram) 분석
[OpenCV] Basic 11 - 이미지 히스토그램 (Histogram) 분석
2024.11.19 -
[OpenCV] Basic 10 - 이미지 색상 변환 (Color Conversion)
[OpenCV] Basic 10 - 이미지 색상 변환 (Color Conversion)
2024.11.16 -
[OpenCV] Basic 8 - 이미지 블러 처리 (Blur)
[OpenCV] Basic 8 - 이미지 블러 처리 (Blur)
2024.11.14 -
[ OpenCV ] Basic 7 - 이미지 자르기 (Crop)
[ OpenCV ] Basic 7 - 이미지 자르기 (Crop)
2022.11.21
댓글을 사용할 수 없습니다.